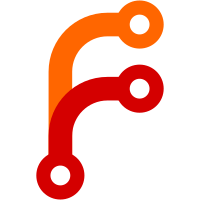
* Add an IntelliJ section to gitignore * Add Android SDK sample project which exercises mentat SDK * Symlink libmentat_ffi.so in Android SDK to the generated --release files * README files for Android SDK and mentat_ffi
98 lines
3.1 KiB
Groovy
98 lines
3.1 KiB
Groovy
apply plugin: 'com.android.library'
|
|
apply plugin: 'kotlin-android'
|
|
apply plugin: 'com.jfrog.bintray'
|
|
// Simply applying this plugin gets bintray to publish a pom file.
|
|
apply plugin: 'com.github.dcendents.android-maven'
|
|
|
|
android {
|
|
compileSdkVersion rootProject.ext.build['compileSdkVersion']
|
|
defaultConfig {
|
|
minSdkVersion rootProject.ext.build['minSdkVersion']
|
|
targetSdkVersion rootProject.ext.build['targetSdkVersion']
|
|
|
|
testInstrumentationRunner "android.support.test.runner.AndroidJUnitRunner"
|
|
}
|
|
buildTypes {
|
|
release {
|
|
minifyEnabled false
|
|
proguardFiles getDefaultProguardFile('proguard-android.txt'), 'proguard-rules.pro'
|
|
}
|
|
}
|
|
|
|
sourceSets {
|
|
androidTest.assets.srcDirs += '../../../../fixtures'
|
|
}
|
|
|
|
// TODO silences:
|
|
// [InvalidPackage]: not included in Android: java.awt. Referenced from com.sun.jna.Native.AWT.
|
|
lintOptions {
|
|
abortOnError false
|
|
}
|
|
}
|
|
|
|
dependencies {
|
|
implementation fileTree(dir: 'libs', include: ['*.jar'])
|
|
androidTestImplementation('com.android.support.test.espresso:espresso-core:2.2.2', {
|
|
exclude group: 'com.android.support', module: 'support-annotations'
|
|
})
|
|
androidTestImplementation 'com.android.support:support-annotations:27.1.1'
|
|
androidTestImplementation 'com.android.support.test:runner:1.0.2'
|
|
androidTestImplementation 'com.android.support.test:rules:1.0.2'
|
|
implementation 'com.android.support:appcompat-v7:27.1.1'
|
|
implementation 'com.android.support.constraint:constraint-layout:1.1.0'
|
|
implementation 'com.android.support:design:27.1.1'
|
|
testImplementation 'junit:junit:4.12'
|
|
implementation "org.jetbrains.kotlin:kotlin-stdlib-jdk7:$kotlin_version"
|
|
implementation 'net.java.dev.jna:jna:4.5.1'
|
|
}
|
|
repositories {
|
|
mavenCentral()
|
|
}
|
|
|
|
// Publishing to jcenter/bintray.
|
|
def libGroupId = properties.libGroupId
|
|
def libRepoName = properties.libRepositoryName
|
|
def libProjectName = properties.libProjectName
|
|
def libProjectDescription = properties.libProjectDescription
|
|
def libUrl = properties.libUrl
|
|
def libVcsUrl = properties.libVcsUrl
|
|
def libLicense = properties.libLicense
|
|
|
|
Properties localProperties = null
|
|
if (project.rootProject.file('local.properties').canRead()) {
|
|
localProperties = new Properties()
|
|
localProperties.load(project.rootProject.file('local.properties').newDataInputStream())
|
|
}
|
|
|
|
version = rootProject.ext.library['version']
|
|
|
|
task sourcesJar(type: Jar) {
|
|
from android.sourceSets.main.java.srcDirs
|
|
classifier = 'sources'
|
|
}
|
|
|
|
artifacts {
|
|
archives sourcesJar
|
|
}
|
|
|
|
|
|
group = libGroupId
|
|
archivesBaseName = libProjectName
|
|
|
|
bintray {
|
|
user = localProperties != null ? localProperties.getProperty("bintray.user") : ""
|
|
key = localProperties != null ? localProperties.getProperty("bintray.apikey") : ""
|
|
|
|
configurations = ['archives']
|
|
pkg {
|
|
repo = libRepoName
|
|
name = libProjectName
|
|
userOrg = "grisha" // Temporary org name until package is on jcenter. Issue #725.
|
|
desc = libProjectDescription
|
|
websiteUrl = libUrl
|
|
vcsUrl = libVcsUrl
|
|
licenses = [libLicense]
|
|
publish = true
|
|
publicDownloadNumbers = true
|
|
}
|
|
}
|