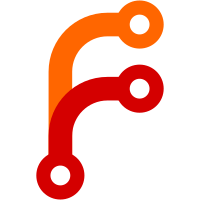
Replacement policies now track the pin count of each page that they interact with. This behavior used to live in bufferHash, and had never been ported to concurrentBufferManager. For various reasons, the pin counts have to be updated atomically with the LRU state. This would be very difficult to do inside of concurrentBufferManager, which sometimes needs to add and remove pages to replacement policies without holding any other latches.
45 lines
1.7 KiB
C
45 lines
1.7 KiB
C
/**
|
|
@file
|
|
|
|
Implements cache replacement policies. Eventually, this could be
|
|
extended to support application specific caching schemes.
|
|
|
|
@todo Stasis used to use LRU-2S. LRU-2S is described in Markatos
|
|
"On Caching Searching Engine Results". (This needs to be
|
|
re-implemented properly.)
|
|
|
|
For now, Stasis uses plain-old LRU. DB-MIN would be an interesting
|
|
extension.
|
|
|
|
If you would like to implement your own caching policy, implement
|
|
the functions below. They are relatively straightforward. Note
|
|
that replacementPolicy implementations do not perform any file I/O
|
|
of their own.
|
|
|
|
The implementation of this module does not need to be threadsafe.
|
|
|
|
*/
|
|
|
|
#include <stasis/doubleLinkedList.h>
|
|
|
|
typedef struct replacementPolicy {
|
|
struct replacementPolicy* (*init)();
|
|
void (*deinit) (struct replacementPolicy* impl);
|
|
void (*hit) (struct replacementPolicy* impl, void * page);
|
|
void*(*getStale)(struct replacementPolicy* impl);
|
|
void*(*remove) (struct replacementPolicy* impl, void * page);
|
|
void*(*getStaleAndRemove)(struct replacementPolicy* impl);
|
|
void (*insert) (struct replacementPolicy* impl, void * page);
|
|
void * impl;
|
|
} replacementPolicy;
|
|
|
|
replacementPolicy * stasis_replacement_policy_lru_init();
|
|
replacementPolicy * lruFastInit(
|
|
struct LL_ENTRY(node_t) * (*getNode)(void * page, void * conf),
|
|
void (*setNode)(void * page,
|
|
struct LL_ENTRY(node_t) * n,
|
|
void * conf),
|
|
intptr_t* (*derefCount)(void *page),
|
|
void * conf);
|
|
replacementPolicy* replacementPolicyThreadsafeWrapperInit(replacementPolicy* rp);
|
|
replacementPolicy* replacementPolicyConcurrentWrapperInit(replacementPolicy** rp, int count);
|