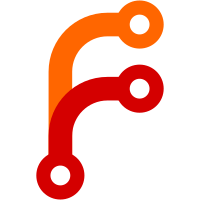
The tests now pass, except that check_merge never terminates (it takes too long) and check_mergelarge still is not passing. For better luck running this version of the code, turn off stasis' concurrent buffer manager. We're doing something bad that leads to deadlocks with the concurrent buffer manager. Another (the same?) bug less-frequently leads to page corruption with the old stasis buffer manager. git-svn-id: svn+ssh://svn.corp.yahoo.com/yahoo/yrl/labs/pnuts/code/logstore@556 8dad8b1f-cf64-0410-95b6-bcf113ffbcfe
104 lines
2.4 KiB
C++
104 lines
2.4 KiB
C++
#include <string>
|
|
#include <vector>
|
|
#include <iostream>
|
|
#include <sstream>
|
|
#include "logstore.h"
|
|
#include "datapage.h"
|
|
#include "logiterators.h"
|
|
#include "merger.h"
|
|
#include <assert.h>
|
|
#include <limits.h>
|
|
#include <math.h>
|
|
#include <pthread.h>
|
|
#include <sys/time.h>
|
|
#include <time.h>
|
|
|
|
#undef begin
|
|
#undef end
|
|
|
|
#include "check_util.h"
|
|
|
|
void insertProbeIter(size_t NUM_ENTRIES)
|
|
{
|
|
unlink("logfile.txt");
|
|
unlink("storefile.txt");
|
|
//data generation
|
|
std::vector<std::string> data_arr;
|
|
std::vector<std::string> key_arr;
|
|
preprandstr(NUM_ENTRIES, data_arr, 10*8192, true);
|
|
preprandstr(NUM_ENTRIES+200, key_arr, 100, true);
|
|
|
|
std::sort(key_arr.begin(), key_arr.end(), &mycmp);
|
|
|
|
removeduplicates(key_arr);
|
|
if(key_arr.size() > NUM_ENTRIES)
|
|
key_arr.erase(key_arr.begin()+NUM_ENTRIES, key_arr.end());
|
|
|
|
NUM_ENTRIES=key_arr.size();
|
|
|
|
if(data_arr.size() > NUM_ENTRIES)
|
|
data_arr.erase(data_arr.begin()+NUM_ENTRIES, data_arr.end());
|
|
|
|
rbtree_t rbtree;
|
|
|
|
int64_t datasize = 0;
|
|
std::vector<pageid_t> dsp;
|
|
for(size_t i = 0; i < NUM_ENTRIES; i++)
|
|
{
|
|
//prepare the key
|
|
datatuple *newtuple = datatuple::create(key_arr[i].c_str(), key_arr[i].length()+1,data_arr[i].c_str(), data_arr[i].length()+1);
|
|
|
|
datasize += newtuple->byte_length();
|
|
|
|
rbtree.insert(newtuple);
|
|
}
|
|
|
|
printf("\nTREE STRUCTURE\n");
|
|
//ltable.get_tree_c1()->print_tree(xid);
|
|
printf("datasize: %lld\n", (long long)datasize);
|
|
|
|
printf("Stage 2: Looking up %d keys:\n", NUM_ENTRIES);
|
|
|
|
int found_tuples=0;
|
|
for(int i=NUM_ENTRIES-1; i>=0; i--)
|
|
{
|
|
//find the key with the given tuple
|
|
int ri = i;
|
|
|
|
//prepare a search tuple
|
|
datatuple *search_tuple = datatuple::create(key_arr[ri].c_str(), key_arr[ri].length()+1);
|
|
|
|
//step 1: look in tree_c0
|
|
|
|
rbtree_t::iterator rbitr = rbtree.find(search_tuple);
|
|
if(rbitr != rbtree.end())
|
|
{
|
|
datatuple *tuple = *rbitr;
|
|
|
|
found_tuples++;
|
|
assert(tuple->keylen() == key_arr[ri].length()+1);
|
|
assert(tuple->datalen() == data_arr[ri].length()+1);
|
|
}
|
|
else
|
|
{
|
|
printf("Not in scratch_tree\n");
|
|
}
|
|
|
|
datatuple::freetuple(search_tuple);
|
|
}
|
|
printf("found %d\n", found_tuples);
|
|
}
|
|
|
|
|
|
|
|
/** @test
|
|
*/
|
|
int main()
|
|
{
|
|
insertProbeIter(250);
|
|
|
|
|
|
|
|
return 0;
|
|
}
|
|
|