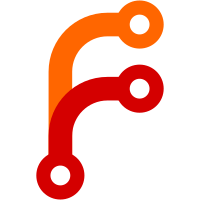
Bugfixes: - Atomically deallocate regions and update the logstore object - Proactively invalidate iterators after each merge (before, it would simply set a not-valid bit. This doesn't work because iterators hold page pins, which breaks force-writes) - Clarify semantics of opening iterators mid-stream: All calls now return iterators that return first key >= the requested one. revalidate() needs the key > the requested one, so it calls peek(), then (if necessary) getnext(). - Add asserts to check that the header is latched at update, and that tuples returned by iterators are strictly monotonically increasing' - Improve error handling in network.h We still get (and terminate on) SIGPIPE. Refactoring: - Add dispatch function to network.h. git-svn-id: svn+ssh://svn.corp.yahoo.com/yahoo/yrl/labs/pnuts/code/logstore@620 8dad8b1f-cf64-0410-95b6-bcf113ffbcfe
41 lines
862 B
C++
41 lines
862 B
C++
/*
|
|
* drop_database.cpp
|
|
*
|
|
* Created on: Feb 23, 2010
|
|
* Author: sears
|
|
*/
|
|
|
|
#include "../tcpclient.h"
|
|
#include "../network.h"
|
|
#include "../datatuple.h"
|
|
|
|
void usage(char * argv[]) {
|
|
fprintf(stderr, "usage %s [host [port]]\n", argv[0]);
|
|
}
|
|
|
|
int main(int argc, char * argv[]) {
|
|
bool ok = true;
|
|
int svrport = 32432;
|
|
char * svrname = "localhost";
|
|
if(argc == 3) {
|
|
svrport = atoi(argv[2]);
|
|
}
|
|
if(argc == 2 || argc == 3) {
|
|
svrname = argv[1];
|
|
}
|
|
if(!ok || argc > 3) {
|
|
usage(argv); return 1;
|
|
}
|
|
|
|
logstore_handle_t * l = logstore_client_open(svrname, svrport, 100);
|
|
|
|
if(l == NULL) { perror("Couldn't open connection"); return 2; }
|
|
|
|
datatuple * ret = logstore_client_op(l, OP_DBG_DROP_DATABASE);
|
|
if(ret == NULL) {
|
|
perror("Drop database failed."); return 3;
|
|
}
|
|
logstore_client_close(l);
|
|
printf("Success\n");
|
|
return 0;
|
|
}
|